Habe mal wieder Lust bekommen etwas Kreatives zu programmieren und stecke zur Zeit etwas fest und zwar bei dem Versuch eine Scheibe aus Punkten zu rotieren.
Hier das Ergebnis und darunter wie es aussehen soll.
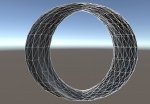
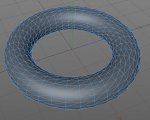
Der Code ist etwas lumpig aber ich wollte schnell etwas auf dem Bildschirm sehen die Zeilen wo etwas rotiert wird sind
45 – 49 bei Test.cs und 144 -146 beim TreeMeshGenerator.cs.
Bin mir mittlerweile nicht mehr sicher ob ich etwas Grundlegend falsch mache oder einfach nur irgendwo einen Fehler habe.
Edit:
Ergibt
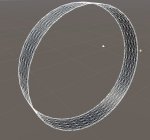
Hier das Ergebnis und darunter wie es aussehen soll.
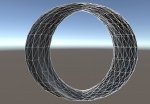
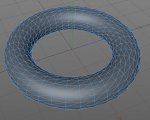
Der Code ist etwas lumpig aber ich wollte schnell etwas auf dem Bildschirm sehen die Zeilen wo etwas rotiert wird sind
45 – 49 bei Test.cs und 144 -146 beim TreeMeshGenerator.cs.
Code:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class TreeMeshGenerator
{
private Vector3[] ringVectors;
private List<int[]> ringTriangles;
public TreeMeshGenerator(int _meshDvision)
{
ringVectors = new Vector3[_meshDvision];
ringTriangles = new List<int[]>();
GenerateLookUpTables(_meshDvision);
}
private void GenerateLookUpTables(int _meshDvishion)
{
//Add Vectors in a ring shape at a range from -1 to +1
for(int i = 0; i < _meshDvishion; i ++)
{
float rad = Mathf.Deg2Rad * 360.0f * ((float) i / (float) (_meshDvishion - 1));
ringVectors[i] = new Vector3(Mathf.Sin(rad),1.0f,Mathf.Cos(rad));
}
/* This will add the trinagles below a example with a mesh dvision of 5
9---8---7---6---5---9 upper ring (node.EndPosition)
| /| /| /| /| /|
| / | / | / | / | / |
|/ |/ |/ |/ |/ |
4---3---2---1---0---4 lower ring (node.StartPosition)
the triangles will be 0-1-5 1-5-6 1-2-6 2-6-7 and so on */
// for(int i = 0; i < _meshDvishion - 1; i ++)
// {
// ringTriangles.Add(new int[]{i,i + 1,_meshDvishion + i});
// //ringTriangles.Add(new int[]{i + 1,_meshDvishion + i, _meshDvishion + i + 1});
// ringTriangles.Add(new int[]{_meshDvishion + i,i + 1, _meshDvishion + i + 1});
//
// }
}
public Mesh BuildMesh(List<Node> _nodeList)
{
List<Vector3> vertices = new List<Vector3>();
foreach(Node node in _nodeList)
{
vertices.AddRange(GetNodeVertices(node,true));
}
for(int i = 0; i < vertices.Count - ringVectors.Length - 1; i ++)
{
ringTriangles.Add(new int[]{i,i + 1,ringVectors.Length + i});
//ringTriangles.Add(new int[]{i + 1,_meshDvishion + i, _meshDvishion + i + 1});
ringTriangles.Add(new int[]{ringVectors.Length + i,i + 1, ringVectors.Length + i + 1});
}
List<int> tmp = new List<int>();
for(int i = 0; i < ringTriangles.Count; i ++)
{
tmp.Add(ringTriangles[i][0]);
tmp.Add(ringTriangles[i][1]);
tmp.Add(ringTriangles[i][2]);
}
Mesh mesh = new Mesh();
mesh.SetVertices(vertices);
mesh.triangles = tmp.ToArray();
return mesh;
// List<Vector3> vertices = new List<Vector3>();
//
// foreach(Node node in _nodeList)
// {
// vertices.AddRange(GetNodeVertices(node));
// }
// Mesh mesh = new Mesh();
// mesh.SetVertices(vertices);
//
// List<int> tmp = new List<int>();
// for(int n = 0; n < _nodeList.Count; n ++)
// for(int i = 0; i < ringTriangles.Count; i ++)
// {
// tmp.Add(ringTriangles[i][0] + (n * ringTriangles.Count));
// tmp.Add(ringTriangles[i][1] + (n * ringTriangles.Count));
// tmp.Add(ringTriangles[i][2] + (n * ringTriangles.Count));
// }
// mesh.triangles = tmp.ToArray();
//
//
// return mesh;
}
private Vector3[] GetNodeVertices(Node node,bool _gotParrent)
{
Vector3[] vertices;
if(!_gotParrent)
{
vertices = new Vector3[ringVectors.Length * 2];
for(int i = 0; i < ringVectors.Length; i ++)
{
//vertices[i] = new Vector3(ringVectors[i].x * node.Width + node.StartPosition.x, node.StartPosition.y,ringVectors[i].z * node.Width + node.StartPosition.z);
vertices[i] = GetTransformedRingVector(ringVectors[i],node.StartPosition,node.Width,node.Rotation,node.Direction);
}
for(int i = 0; i < ringVectors.Length; i ++)
{
//vertices[i + ringVectors.Length] = new Vector3(ringVectors[i].x * node.Width + node.EndPosition.x, node.EndPosition.y,ringVectors[i].z * node.Width + node.EndPosition.z);
vertices[i] = GetTransformedRingVector(ringVectors[i],node.EndPosition,node.Width,node.Rotation,node.Direction);
}
return vertices;
}
vertices = new Vector3[ringVectors.Length];
for(int i = 0; i < ringVectors.Length; i ++)
{
//vertices[i] = new Vector3(ringVectors[i].x * node.Width + node.EndPosition.x, node.EndPosition.y,ringVectors[i].z * node.Width + node.EndPosition.z);
vertices[i] = GetTransformedRingVector(ringVectors[i],node.EndPosition,node.Width,node.Rotation,node.Direction);
}
return vertices;
}
// private Vector3[] GetNodeVertices(Node node)
// {
// Vector3[] vertices = new Vector3[ringVectors.Length];
// for(int i = 0; i < ringVectors.Length; i ++)
// {
// vertices[i + ringVectors.Length] = new Vector3(ringVectors[i].x * node.Width + node.EndPosition.x, node.EndPosition.y,ringVectors[i].z * node.Width + node.EndPosition.z);
//
// }
// return vertices;
// }
private Vector3 GetTransformedRingVector(Vector3 _ringVector,Vector3 _position,float _width,Quaternion _rotation,Vector3 _direction)
{
Vector3 a = new Vector3(_ringVector.x * _width + _position.x, _position.y,_ringVector.z * _width + _position.z);
Vector3 b = (a - _position);
a = new Vector3(b.x,b.y * Mathf.Cos(_direction.y * Mathf.Deg2Rad),b.z) + _position;
return a;
}
}
Code:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class test : MonoBehaviour
{
Mesh mesh;
// Use this for initialization
void Start ()
{
GrowSomeStuff();
// List<Node> nodeList = new List<Node>();
// nodeList.Add(new Node());
//
// nodeList.Add(new Node());
// nodeList[1].StartPosition = nodeList[1].EndPosition;
// nodeList[1].EndPosition = new Vector3(3,5,3);
// float angle = Vector3.Angle(nodeList[1].StartPosition,nodeList[1].EndPosition);
// nodeList[1].Rotation = Quaternion.Euler(0,angle,0);
// nodeList.Add(new Node());
// nodeList[2].StartPosition = nodeList[1].EndPosition;
// nodeList[2].EndPosition = new Vector3(13,15,13);
// angle = Vector3.Angle(nodeList[2].StartPosition,nodeList[2].EndPosition);
// nodeList[1].Rotation = Quaternion.Euler(0,angle,0);
// nodeList.Add(new Node());
// nodeList[3].StartPosition = nodeList[2].EndPosition;
// nodeList[3].EndPosition = new Vector3(20,15,20);
// angle = Vector3.Angle(nodeList[3].StartPosition,nodeList[3].EndPosition);
// nodeList[3].Rotation = Quaternion.Euler(0,angle,0);
}
public void GrowSomeStuff()
{
TreeMeshGenerator meshGen = new TreeMeshGenerator(15);
List<Node> nodeList = new List<Node>();
nodeList.Add(new Node());
float distance = 2;
Node node;
for(int i = 0; i < 36; i ++)
{
node = new Node();
node.StartPosition = nodeList[i].EndPosition;
node.Direction = new Vector3(0,nodeList[i].Direction.y + 10 ,0);
node.EndPosition = new Vector3(((Mathf.Cos (node.Direction.x * Mathf.Deg2Rad)* Mathf.Sin (node.Direction.y * Mathf.Deg2Rad)) * distance) + node.StartPosition.x,
(Mathf.Cos (node.Direction.y * Mathf.Deg2Rad) * distance) + node.StartPosition.y,
((Mathf.Sin (node.Direction.x * Mathf.Deg2Rad) * Mathf.Sin (node.Direction.y * Mathf.Deg2Rad)) * distance) + node.StartPosition.z);
node.Direction = new Vector3(0,node.Direction.y ,0);
nodeList.Add(node);
}
mesh = meshGen.BuildMesh(nodeList);
mesh.RecalculateNormals();
this.GetComponent<MeshFilter>().mesh = mesh;
for(int i = 0; i < mesh.triangles.Length - 1; i ++)
{
Debug.DrawLine(mesh.vertices[mesh.triangles[i]],mesh.vertices[mesh.triangles[i+1]],Color.white,60.0f);
}
}
}
Bin mir mittlerweile nicht mehr sicher ob ich etwas Grundlegend falsch mache oder einfach nur irgendwo einen Fehler habe.
Edit:
Code:
a = new Vector3(b.x * Mathf.Sin(_direction.z * Mathf.Deg2Rad),b.y * Mathf.Cos(_direction.y * Mathf.Deg2Rad),b.z* Mathf.Cos(_direction.z * Mathf.Deg2Rad)) + _position;
Ergibt
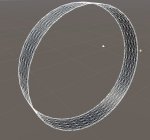
Zuletzt bearbeitet: